iOS
This page details how to add the ReadyRemit SDK Package to your Project for iOS
Step 1 - Import the SDK
1.1 - Using Swift Package Manager
- Add the ReadyRemitSDK package to your Package.swift
.package(
url: "https://github.com/BrightwellPayments/readyremit-sdk-ios",
.branch("9.0")
)
- Update your packages running the command
swift package update
- Alternativelly you can import it directly via XCode,
- In Xcode's top menu, go to
File > Add Packages....
- In the dialog that appears, enter the URL of the repository in the search bar:
https://github.com/BrightwellPayments/readyremit-sdk-ios
- Select Branch from the dependency rules
- Enter
9.0
(or the desired version) as the branch name. - Click Add Package.
- Add the Package to Your Targets
1.2 - Using Cocoapods
- Open the Podfile in a text editor.
- Add the following line, replacing 9.0 with the desired version of the SDK:
pod 'ReadyRemitSDK', :git => 'https://github.com/BrightwellPayments/readyremit-sdk-ios.git', :branch => '9.0'
- Install the pod running
pod install
1.3 - Using binary
- Download the
ReadyRemitSDK.zip
from here. - Unzip the file
- Create a
Frameworks
folder under your root project folder - Paste the unzipped
ReadyRemitSDK.xcframework
into the newly createdFrameworks
folder
Add the SDK to your project
- Open your Project in XCode and go to the Project -> General -> Frameworks, Libraries, and Embedded Content.
- Drag and drop the downloaded SDK into the "Frameworks, Libraries, and Embedded Content" section of the settings.
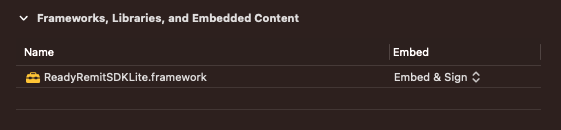
Install required libraries
- Download the
VisaSensoryBranding.zip
file from Github. - Extract the contents of the zip file and add the extracted library folder to the newly created "Frameworks" directory.
- In XCode select your main target in the "Project Navigator" window and switch to the "General" tab.
- Under "Frameworks, Libraries and Embedded Content" drag and drop the
VisaSensoryBranding.xcframework
folder
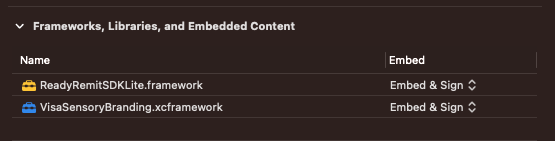
Step 2 - Implement ReadyRemit Delegate
Open the View Controller you will be launching the ReadyRemit SDK from, and add the ReadyRemitDelegate protocol to the ViewController class definition. (You will need to import the ReadyRemit SDK.)
You will want to conform it to the protocol by adding two functions.
The first function gets an Auth Token. This function will have two callbacks. One is to run if the call back is successful, and a second is a failure.
Security Warning
The Auth Token can be used to view users' personal information and bank information. Because of this, you should never store it in long-term storage.
//ReadyRemitSDK
import ReadyRemitSDK
class ViewController: UIViewController, ReadyRemitDelegate {
...
func onAuthTokenRequest(success: @escaping (String) -> Void,
failure: @escaping () -> Void) {
// If token is available
let authToken = "ACCESS_TOKEN_FROM_SERVER"
DispatchQueue.main.async {
success(authToken)
}
..
// If token is unavailable
DispatchQueue.main.async {
failure()
}
}
}
The second function is the onSubmitTransfer function. You should make an API call to your server with the TransferRequest data through this function. This gives you a TransferId in return which you will give to the SDK.
//ReadyRemitSDK
import ReadyRemitSDK
class ViewController: UIViewController, ReadyRemitDelegate {
...
func onSubmitTransfer(transferRequest: ReadyRemit.TransferRequest,
success: @escaping (String) -> Void,
failure: @escaping (String, String) -> Void) {
// Make an API call to your server with the TransferReqeust data
// Expect a transferId to be returned
let transferId = "abc"
DispatchQueue.main.async {
success(transferId)
}
..
// If token is unavailable
let errorMessage = "error message from server"
let errorCode = "error code from server"
DispatchQueue.main.async {
failure(errorMessage, errorCode)
}
}
}
Note on API Endpoints
As of May 1, 2024, Apple will require all apps submitted to the App Store to include a detailed declaration of API usage in their privacy manifest files. To comply with this requirement, developers must add an
NSPrivacyAccessedAPITypes
array to theInfo.plist
file of the app. This array should contain dictionary entries for each API, including ones for ReadyRemit, that accesses user or device data, specifying the category of the API and providing a clear, approved reason for its usage. These reasons must align with Apple's guidelines on privacy transparency. It is crucial for developers to review their code for API usage in categories such as SystemBootTime, FileTimestamp, UserDefaults, and DiskSpace, and to document their necessity and purpose.
Step 3 - Initialize your SDK
The last step is to initialize the SDK.
Note on UINavigationController
The ReadyRemit SDK initialization requires that the ViewController launching the SDK has a parent NavigationController available.
guard let controller = self.navigationController else {
// ERROR handling in case the navigation controller isn't available
return;
}
ReadyRemit.shared.languageSelected("en_US") // Value can be "en_US" or "es_MX"
ReadyRemit.shared.environment = .sandbox // Value can be .sandbox or .production
ReadyRemit.shared.launch(inNavigation: controller, delegate: self) {}
(Optional) Style the ReadyRemit SDK
You can style the SDK following this example
- Colors
let colors = createColors()
colors.primary = UIColor(lightHex: "#934AE0", darkHex: "#844FBB");
colors.primaryLight = UIColor(lightHex: "#F3EDFB", darkHex: "#2B2332");
colors.buttonColor = UIColor(lightHex: "#743FC9", darkHex: "#743FC9");
colors.buttonText = UIColor(lightHex: "#FFFFFF", darkHex: "#FFFFFF");
colors.text = UIColor(lightHex: "#0E0F0C", darkHex: "#E3E3E3");
colors.textSecondary = UIColor(lightHex: "#454545", darkHex: "#B0B0B0");
colors.foreground = UIColor(lightHex: "#FFFFFF", darkHex: "#1F1F1F");
colors.background = UIColor(lightHex: "#F3F4F6", darkHex: "#111111");
colors.icon = UIColor(lightHex: "#444444", darkHex: "#7E7E7E");
colors.inputLine = UIColor(lightHex: "#858585", darkHex: "#505050");
colors.divider = UIColor(lightHex: "#E2E2E2", darkHex: "#313131");
colors.success = UIColor(lightHex: "#008761", darkHex: "#008761");
colors.danger = UIColor(lightHex: "#AA220F", darkHex: "#ED7083");
colors.dangerLight = UIColor(lightHex: "#F4E9E7", darkHex: "#533338");
ReadyRemit.shared.appearance = ReadyRemitAppearance(colors: colors)
- Fonts
Utilizing Custom Fonts
To employ a custom font, please make sure it is correctly installed within your application. Also, ensure that it includes semi-bold and bold variations for a complete experience.
ReadyRemitFontScheme.defaultFamily = "Arial"
(Optional) Closing ReadyRemitSDK
If you wish to gracefully close the ReadyRemitSDK at any point in your code, you can achieve this with the following method:
func closeReadyRemitSDK() {
ReadyRemit.shared.close()
}
(Optional) Inactivity Detector
If you prefer to automatically close the ReadyRemitSDK when your users are inactive, you can use the following snippet. Place it in your activity where you launch the SDK.
import UIKit
class YourViewController : UIViewController() {
@IBAction private func onSendMoney(_ sender: Any) {
guard let controller = self.navigationController else {
// ERROR handling in case the navigation controller isn't available
return
}
// Launch ReadyRemitSDK
ReadyRemit.shared.environment = .sandbox // Value can be .sandbox or .production
ReadyRemit.shared.launch(inNavigation: controller, delegate: self) {}
// Set inactivity timeout (e.g., 60 seconds)
ReadyRemit.shared.close(afterSeconds: 60)
}
}
(For KYC users only) Enable Camera Permission
If you wish to use the ReadyRemitSDK with the KYC feature enabled, you will need to ask your users for Camera Permission, here is how you can do that.
- In XCode, open your info.plist file and add a new key for
Privacy - Camera Usage Description
with a value explaining why you need to use the camera. We recommend the following text:
Verify my identity per KYC standards before I can start sending money to recipients

Updated 7 months ago