ReactNative - iOS
This guide will help you integrate the ReadyRemitSDK into your React Native developed iOS projects.
The ReadyRemitSDK is supported from iOS 14 to iOS 17.
Step 1 - Download the SDK
Version specification
You will find two versions of ReadyRemitSDK
• ReadyRemitSDK.zip - This is the full version that includes the KYC feature
• ReadyRemitSDKLite.zip - This is the lite version, that does not include the KYC feature
You should implement only one of these into your project
- Download the
ReadyRemitSDK.zip
orReadyRemitSDKLite.zip
from here. - Unzip the file
- Create a
Frameworks
folder under your root ios project folder (myProject/ios) - Paste the unzipped
ReadyRemitSDK.xcframework
orReadyRemitSDKLite.xcframework
on the newly createdFrameworks
folder
Step 2 - Add the SDK to your project
- Open your Project in XCode and go to the Project -> General -> Frameworks, Libraries, and Embedded Content.
- Drag and drop the downloaded SDK into the "Frameworks, Libraries, and Embedded Content" section of the settings.
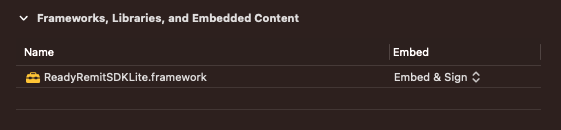
Step 3 - Install required libraries
- Download the
VisaSensoryBranding.zip
file from Github. - Extract the contents of the zip file and add the extracted library folder to the newly created "Frameworks" directory.
- In XCode select your main target in the "Project Navigator" window and switch to the "General" tab.
- Under "Frameworks, Libraries and Embedded Content" drag and drop the
VisaSensoryBranding.xcframework
folder
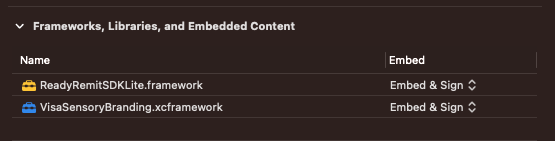
Step 4 - Create the ViewController
ReadyRemit requires a ViewController to display. In the root directory of your iOS project in Xcode, create the following 2 files:
Imports
You should import only one version of ReadyRemitSDK
#import "ReadyRemitSDK/ReadyRemitSDK.h"
- for the full version that contains embedded KYC feature
#import "ReadyRemitSDKLite/ReadyRemitSDKLite-Swift.h"
- for the lite versions that do not contains KYC feature
#import <UIKit/UIkit.h>
//ReadyRemitSDK
#import "ReadyRemitSDK/ReadyRemitSDK.h"
//ReadyRemitSDKLite
#import "ReadyRemitSDKLite/ReadyRemitSDKLite-Swift.h"
@interface ReadyRemitViewController : UIViewController
@property (nonatomic, assign) id<ReadyRemitDelegate> delegate;
@property (nonatomic, assign) NSString* language;
@property (nonatomic, assign) ReadyRemitEnvironment environment;
@property (nonatomic, strong) ReadyRemitAppearance* appearance;
@end
#import "ReadyRemitViewController.h"
//ReadyRemitSDK
#import "ReadyRemitSDK/ReadyRemitSDK.h"
//ReadyRemitSDKLite
#import "ReadyRemitSDKLite/ReadyRemitSDKLite-Swift.h"
@interface ReadyRemitViewController ()
@property (weak, nonatomic) ReadyRemit* readyRemit;
@property (nonatomic, copy) void (^launchCompletion)(void);
@property (nonatomic, copy) void (^onDismiss)(void);
@end
@implementation ReadyRemitViewController
- (void) viewDidLoad {
[super viewDidLoad];
_launchCompletion = ^{ NSLog(@"ObjC: ReadyRemit launched."); };
_onDismiss = ^{ [[self navigationController] dismissViewControllerAnimated: YES completion:nil]; };
_readyRemit = [ReadyRemit shared];
_readyRemit.appearance = _appearance;
_readyRemit.environment = _environment;
[_readyRemit languageSelected:_language];
[_readyRemit launchObjcInNavigation:[self navigationController]
delegate: _delegate
onLaunch: _launchCompletion
onDismiss: _onDismiss];
}
@end
Step 5 - Create the Bridge
In React Native, a "Bridge" is used to facilitate communication and interaction between your React Native code and your native modules. (See the React Native documentation to learn more about this.)
In the root of your iOS project in Xcode, create the following 2 files:
#import <React/RCTBridgeModule.h>
#import <React/RCTEventEmitter.h>
//ReadyRemitSDK
#import "ReadyRemitSDK/ReadyRemitSDK.h"
//ReadyRemitSDKLite
#import "ReadyRemitSDKLite/ReadyRemitSDKLite-Swift.h"
@interface RCTReadyRemitModule : RCTEventEmitter <RCTBridgeModule, ReadyRemitDelegate> {
void (^_authSuccessCallback)(NSString *token);
void (^_transferSuccessCallback)(NSString *token);
void (^_transferFailCallback)(NSString *error, NSString *errorMessage);
}
@end
#import "RCTReadyRemitModule.h"
#import "AppDelegate.h"
#import "ReadyRemitViewController.h"
//ReadyRemitSDK
#import "ReadyRemitSDK/ReadyRemitSDK.h"
//ReadyRemitSDKLite
#import "ReadyRemitSDKLite/ReadyRemitSDKLite-Swift.h"
@implementation RCTReadyRemitModule
- (NSArray<NSString *> *)supportedEvents
{
return @[@"READYREMIT_AUTH_TOKEN_REQUESTED", @"READYREMIT_TRANSFER_SUBMITTED", @"SDK_CLOSED"];
}
RCT_EXPORT_MODULE();
RCT_EXPORT_METHOD(setAuthToken: (NSString *)token :(NSString *)errorCode) {
_authSuccessCallback(token);
}
RCT_EXPORT_METHOD(setTransferId: (NSString *)transferId :(NSString *)errorCode :(NSString *)errorMessage) {
if (transferId != nil && transferId.length > 0) {
_transferSuccessCallback(transferId);
} else {
_transferFailCallback(errorMessage, errorCode);
}
}
RCT_EXPORT_METHOD(launch: (NSString *)environment :(NSString *)language :(NSDictionary*)styles) {
dispatch_async(dispatch_get_main_queue(), ^(void) {
ReadyRemitViewController *readyRemitViewController = [[ReadyRemitViewController alloc] init];
UINavigationController *navigationController = [[UINavigationController alloc] initWithRootViewController:readyRemitViewController];
[readyRemitViewController setModalPresentationStyle: UIModalPresentationOverCurrentContext];
[navigationController setModalPresentationStyle: UIModalPresentationOverCurrentContext];
ReadyRemitFontScheme.defaultFamily = [styles valueForKeyPath:@"fonts.default.family"];
if ([environment isEqual:@"PRODUCTION"]) {
readyRemitViewController.environment = ReadyRemitEnvironmentProduction;
} else {
readyRemitViewController.environment = ReadyRemitEnvironmentSandbox;
}
ReadyRemitAppearance *appearance = [[ReadyRemitAppearance alloc] initWithStyles: styles];
readyRemitViewController.language = language;
readyRemitViewController.delegate = self;
readyRemitViewController.appearance = appearance;
UIWindow *window = [UIApplication sharedApplication].delegate.window;
[window.rootViewController presentViewController:navigationController animated:YES completion:nil];
});
}
- (void) onAuthTokenRequestWithSuccess:(void (^)(NSString * _Nonnull))success failure:(void (^)(void))failure {
_authSuccessCallback = [success copy];
[self sendEventWithName:@"READYREMIT_AUTH_TOKEN_REQUESTED" body:@{ }];
}
- (void) onSubmitTransferWithTransferRequest:(TransferRequest *)transferRequest success:(void (^)(NSString * _Nonnull))success failure:(void (^)(NSString * _Nonnull, NSString * _Nonnull __strong))failure {
_transferSuccessCallback = [success copy];
_transferFailCallback = [failure copy];
[self sendEventWithName:@"READYREMIT_TRANSFER_SUBMITTED" body:[transferRequest toJSON]];
}
- (void) onSDKClose {
[self sendEventWithName:@"SDK_CLOSED" body:@{ }];
}
@end
(Optional) Closing ReadyRemitSDK
If you wish to gracefully close the ReadyRemitSDK at any point in your code, please:
- Add this code to your RCTReadyRemitModule.m
RCT_EXPORT_METHOD(close) {
[[ReadyRemit shared] close];
}
- Call this function in your React Native project:
ReadyRemitModule.close();
(Optional) Inactivity Detector
If you prefer to automatically close the ReadyRemitSDK when your users are inactive, you can add the following snippet into your ReadyRemitModule.kt
- (void) viewDidLoad {
[super viewDidLoad];
_launchCompletion = ^{ NSLog(@"ObjC: ReadyRemit launched."); };
_onDismiss = ^{ [[self navigationController] dismissViewControllerAnimated: YES completion:nil]; };
_readyRemit = [ReadyRemit shared];
_readyRemit.appearance = _appearance;
_readyRemit.environment = _environment;
[_readyRemit languageSelected:_language];
// Launch ReadyRemitSDK
[_readyRemit launchObjcInNavigation:[self navigationController]
delegate: _delegate
onLaunch: _launchCompletion
onDismiss: _onDismiss];
// Set inactivity timeout (e.g., 60 seconds)
[_readyRemit closeAfterSeconds:60];
}
(For KYC users only) Implement KYC
If you wish to use the ReadyRemitSDK with the KYC feature enabled, these are the steps to implement required additional libraries.
- Download
KYC_Frameworks.zip
file from Github. - Extract the contents of the zip file and add all the extracted library folders to the newly created "Frameworks" directory.
- In XCode select your main target in the "Project Navigator" window and switch to the "General" tab.
- Under "Frameworks, Libraries and Embedded Content" drag and drop the all new pasted folders
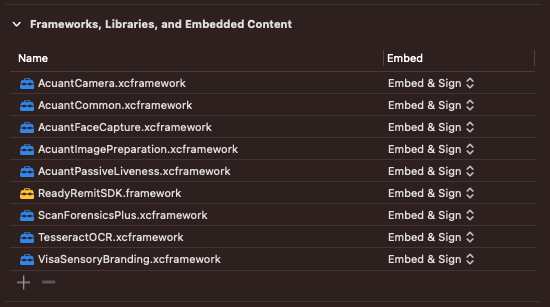
- In XCode, open your info.plist file and add a new key for
NSCameraUsageDescription
with a value explaining why you need to use the camera. We recommend the following text:
Verify my identity per KYC standards before I can start sending money to recipients
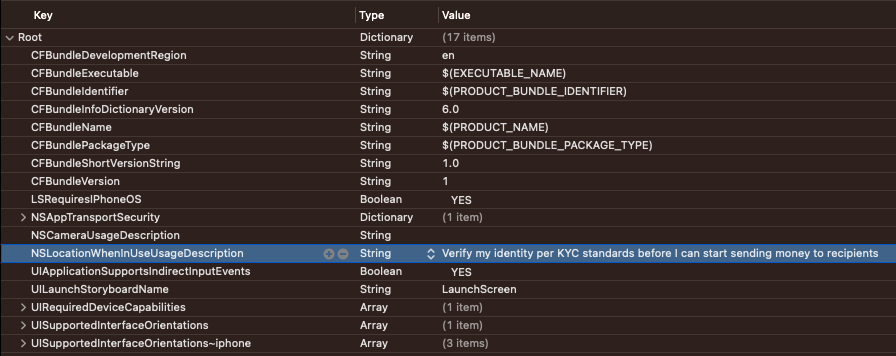
- Add new strings localized strings to your project. Follow Apple's guide for supporting languages and regions in iOS applications.
"acuant_camera_align" = "ALIGN";
"acuant_camera_manual_capture" = "ALIGN & TAP";
"acuant_camera_move_closer" = "MOVE CLOSER";
"acuant_camera_hold_steady" = "HOLD STEADY";
"acuant_camera_capturing" = "CAPTURING";
"acuant_camera_outside_view" = "TOO CLOSE!";
"acuant_face_camera_initial" = "Align face to start capture";
"acuant_face_camera_face_too_close" = "Too Close! Move Away";
"acuant_face_camera_face_too_far" = "Move Closer";
"acuant_face_camera_face_has_angle" = "Face has Angle. Do not tilt";
"acuant_face_camera_face_not_in_frame" = "Move in Frame";
"acuant_face_camera_face_moved" = "Hold Steady";
"acuant_face_camera_capturing_2" = "Capturing\n2...";
"acuant_face_camera_capturing_1" = "Capturing\n1...";
"idology_header_title" = "Please capture the front of the ID";
"idology_retry" = "Retry";
"idology_cancel" = "Cancel";
"idology_confirm" = "Confirm";
"idology_captureBack" = "If you are satisfied please confirm and capture the back of the document";
"idology_captureSelfie" = "If you are satisfied please confirm and please take a selfie";
"idology_thankYou" = "If you are satisfied with all the images, please confirm";
"idology_start" = "Start";
"acuant_camera_align" = "CENTRAR";
"acuant_camera_manual_capture" = "CENTRE Y TOQUE LA PANTALLA";
"acuant_camera_move_closer" = "ACÉRQUESE";
"acuant_camera_hold_steady" = "MANTÉNGASE FIRME";
"acuant_camera_capturing" = "CAPTURANDO";
"acuant_camera_outside_view" = "¡MUY CERCA!";
"acuant_face_camara_initial" = "Centre su rostro para tomar la foto";
"acuant_face_camera_face_too_close" = "¡Muy cerca! Retroceda";
"acuant_face_camera_face_too_far" = "Acérquese";
"acuant_face_camera_face_has_angle" = "Su rostro está inclinado. Por favor no lo incline";
"acuant_face_camera_face_not_in_frame" = "Muévase dentro del marco";
"acuant_face_camera_face_moved" = "Mantenga el teléfono estable";
"acuant_face_camera_capturing_2" = "Capturando\n2...";
"acuant_face_camera_capturing_1" = "Capturando\n1...";
"idology_header_title" = "Capture el frente de la identificación";
"idology_retry" = "Reintentar";
"idology_cancel" = "Cancelar";
"idology_confirm" = "Confirmar";
"idology_captureBack" = "Si está satisfecho, confirme y capture el dorso del documento";
"idology_captureSelfie" = "Si está satisfecho, confirme y tome una foto de su rostro";
"idology_thankYou" = "Si está satisfecho con las imágenes, por favor confirme";
"idology_start" = "Comenzar";
Updated 3 months ago